curses
There are situations where one might want to develop a user interface without making use of an X server, especially where the user might be limited to the command line while requiring some form of application menu. The curse API is provided as one of the core Python modules, and rendering a GUI doesn't require the maintenance of multiple resource files or the coding of logic as GUI element handlers. Overall it's a more portable and lightweight alternative to using PythonCard. The following is an example of a UI rendered using the curses API:
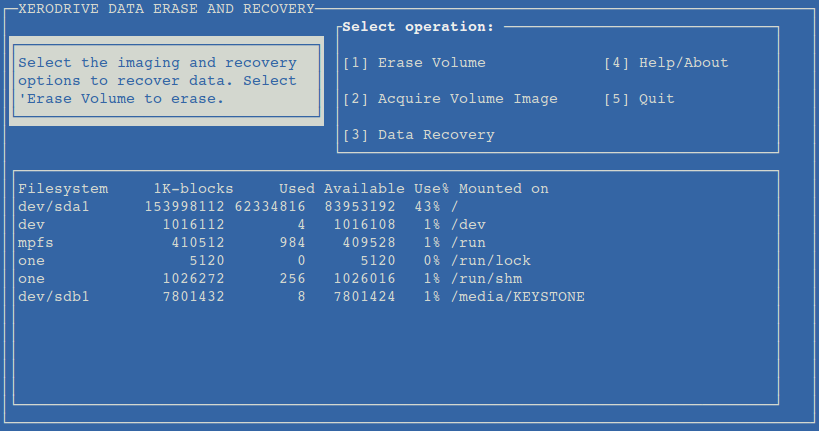
br>
The following lines imports the curses API and initialises the main UI are:
import curses
myscreen = curses.initscr()
Next, we might set the UI border and position for a UI element:
myscreen.border(0);
myscreen.addstr(5,5, "Hello World")
input = myscreen.getstr(12, 20, 50)
myscreen.refresh()
curses.endwin()
IBM developerWorks: Curses programming
distutils
Python Module Distribution Utilities. Used for distributing, packaging and installing Python modules. A
setup.py file is read, which specifies the modules to install on execution.
An alternative is
setuptools.
The distutils module includes:
- ccompiler
- cmd
- core
- extension
hashlib
Used for hashing files or text input. The following algorithms are included by default:
- md5()
- sha1()
- sha224()
- sha256()
- sha384()
- sha512()
Example:
hashlib.sha256(txtPassword).hexdigest()
PyCrypto could also be used for hashing input. e.g.
from Crypto.Hash import SHA256
SHA256.new('abc').hexdigest()
PythonCard
For creating GUI Python applications. The following can be imported:
For example:
import PythonCard
from PythonCard import model
The GUI element position, sizes and colours are defined in the associated
.rsrc.py source file.
Use the following to declare the GUI's background from the model
class: class MyBackground(model.Background):
To capture events, or make the GUI event-driven, we can use
(self, event)
, referencing an element in the
rsrc.py file. For example, if we have defined an element '
cmdButton', we read a mouse click with:
on_cmdEncrypt_mouseClick(self, event)
PythonCard @ SourceForge.net
PyCrypto
This is the Python Cryptography Toolkit. The commonly-used crypto module for Python.
An operation to encrypt text can theoretically be simple, with certain text lengths and cipher modes. For example, to use DES in ECB mode to encrypt an eight character string:
from Crypto.Cipher import DES
des = DES.new('01234567', DES.MODE_ECB)
plainText = 'abcdefgh'
cipherText = des.encrypt(plainText)
print(cipherText)
In order to use a more secure cipher block chaining mode, we need to specify a random initial value (IV) and declare two instances of the cipher function since random values would be generated for both:
from Crypto.Cipher import DES
from Crypto import Random
iv = Random.get_random_bytes(8)
desInstance1 = DES.new('01234567', DES.MODE_CFB, iv)
desInstance2 = DES.new('01234567', DES.MODE_CFB, iv)
text = 'abcdefghijklmnop'
cipher_text = desInstance1.encrypt(text)
print(cipher_text)
desInstance2.decrypt(cipher_text)
PyCrypto could also be used for public key cryptography operations. First, we could use it to generate a private key from which the public key could also be derived:
from Crypto.PublicKey import RSA
from Crypto import Random
randomOut = Random.new().read
privateKey = RSA.generate(1024, randomOut)
print(privateKey)
To encrypt data:
publicKey = key.publickey()
cipherText = publicKey.encrypt('abcdefgh', 32)
print(cipherText)
And to decrypt data:
privateKey.decrypt(cipherText)
Site here...