The official documentation is pretty dry and hard to follow, so I decided to create a simple client to get a better understanding of how it's implemented. I recommend reading Ewout Kramer's presentation material on this.
It appears that FHIR mandates HTTP as the transport method, with the repositories of patient records being queried using REST.
There are four layers to this:
- REST
- Documents
- Messages
- Services
The URI structure for a request is:
http://[endpoint]/[resource type]/[identifier]
The patient record should be returned by the server as an XML or JSON document.
Note: the FHIR documentation refers almost always to version 3 HL7 messages, and not the older segmented version. In HL7, we see data objects communicated as documents. With FHIR, data objects are accessed as services - this makes it more appropriate for REST, with each object being requested having its own URI.
A 'resource' in FHIR is the smallest discreet data object that could be communicated. It's described as the 'smallest unit of transaction', which means it would be an HL7 message.
It is the quantum of FHIR. In fact, a resource is basically an XML file containing the information about a subject, which could be a patient, a report, etc. Within a resource are components - a component has no meaning or context outside the resource. Unlike resources, components cannot be accessed directly.
Within a resource there are also fields, which are referred to as 'composite datatypes', and they're so-called because they might contain multiple sub-items of different types, for example, 'identifier' might be a composite type based on 'varchar' or 'int', and 'Address' might be a composite of '
houseNumber(int)' and '
streetName(varchar)'. Each of these sub-items is referred to as a 'primitive'.
Creating a Client Application Using the C# .NET API
When implementing in a C# .NET project, you'll require the Hl7.Fhir.DSTU2 package through NuGet.
Add the following import statements:
using Hl7.Fhir;
using Hl7.Fhir.Rest;
using Hl7.Fhir.Model;
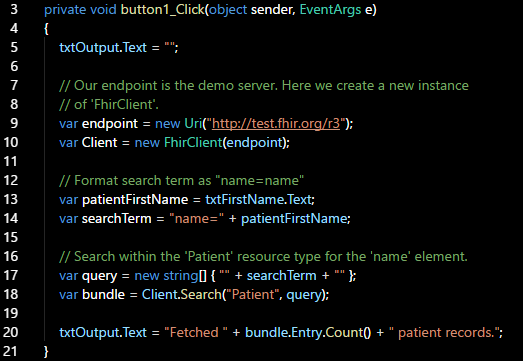
Executing the program:
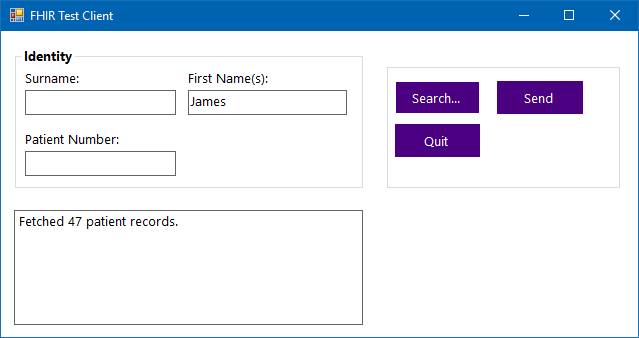
In WireShark we can see the requests and response.
GET /r3/Patient?name=Rob HTTP/1.1
User-Agent: .NET FhirClient for FHIR 1.0.2
Host: test.fhir.org
What we get back is a large XML message containing FHIR resource elements for each patient record discovered on the server.
We should also be able to extract that data to display in the Windows form application.
Creating a Patient Record
The next feature I added was to enable the user to create a very basic patient record.
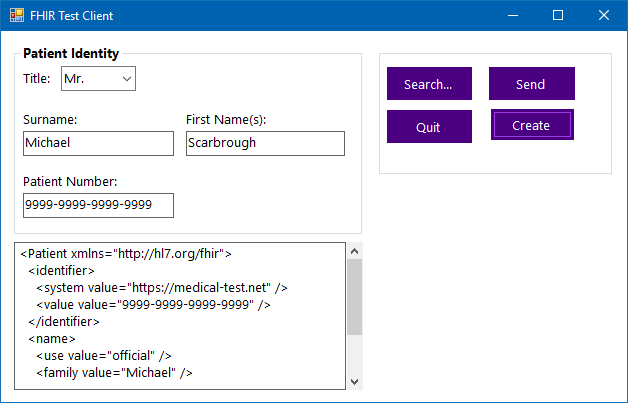
Create a new instance of the patient object from the HL7 FHIR model:
var MyPatient = new Hl7.Fhir.Model.Patient();
var PatientName = new Hl7.Fhir.Model.HumanName();
PatientName.Use = Hl7.Fhir.Model.HumanName.NameUse.Official;
Next I'm simply taking the variables
patientFirstName,
patientSurname,
patientTitle and
patientNumber from Windows Forms elements:
PatientName.Prefix = new string[] { patientTitle };
PatientName.Given = new string[] { patientFirstName };
PatientName.Family = new string[] { patientSurname };
Added to the record are the System and Value objects from the Identifier model.
var PatientIdentifier = new Hl7.Fhir.Model.Identifier();
PatientIdentifier.System = "https://medical-test.net";
PatientIdentifier.Value = patientNumber;
MyPatient.Identifier = new List();
MyPatient.Identifier.Add(PatientIdentifier);
The next several lines is the serialisation.
string xml = Hl7.Fhir.Serialization.FhirSerializer.SerializeResourceToXml(MyPatient);
XDocument xDoc = XDocument.Parse(xml);
txtOutput.Text = xDoc.ToString();
Note the serialisation options. The output can be formatted as JSON as well as XML.
References
BALTUS, M. 2014. FHIR API for .NET Programmers.
FHIR Developer Days. [PDF].
https://www.slideshare.net/DevDays2014/04-b-fhir-api-for-net-mirjam. (7th July 2017).
HEESCH, S. 2014. FHIR Client (Visual Studio 2013).
Windows Dev Center. [WWW].
https://code.msdn.microsoft.com/windowsdesktop/Client-for-HL7-FHIR-server-0709be0b/. (7th July 2017).
HL7.ORG. 2011. Base Resource Definitions.
FHIR Release 3. [WWW].
http://hl7.org/fhir/resource.html">http://hl7.org/fhir/resource.html. (7th July 2017).
KRAMER, E. 2014. HL7 FHIR Training Course - Morning.
HL7 International. [PDF].
https://www.slideshare.net/ewoutkramer/fhir-tutorial-morning. (10th July 2017).
KRAMER, E. 2014. HL7 FHIR Training Course - Afternoon.
HL7 International. [PDF].
https://www.slideshare.net/ewoutkramer/afternoon-32654292. (10th July 2017).